Proxima
2D
TypeScript
Node.js
HTML5
Pixi.js
Colyseus
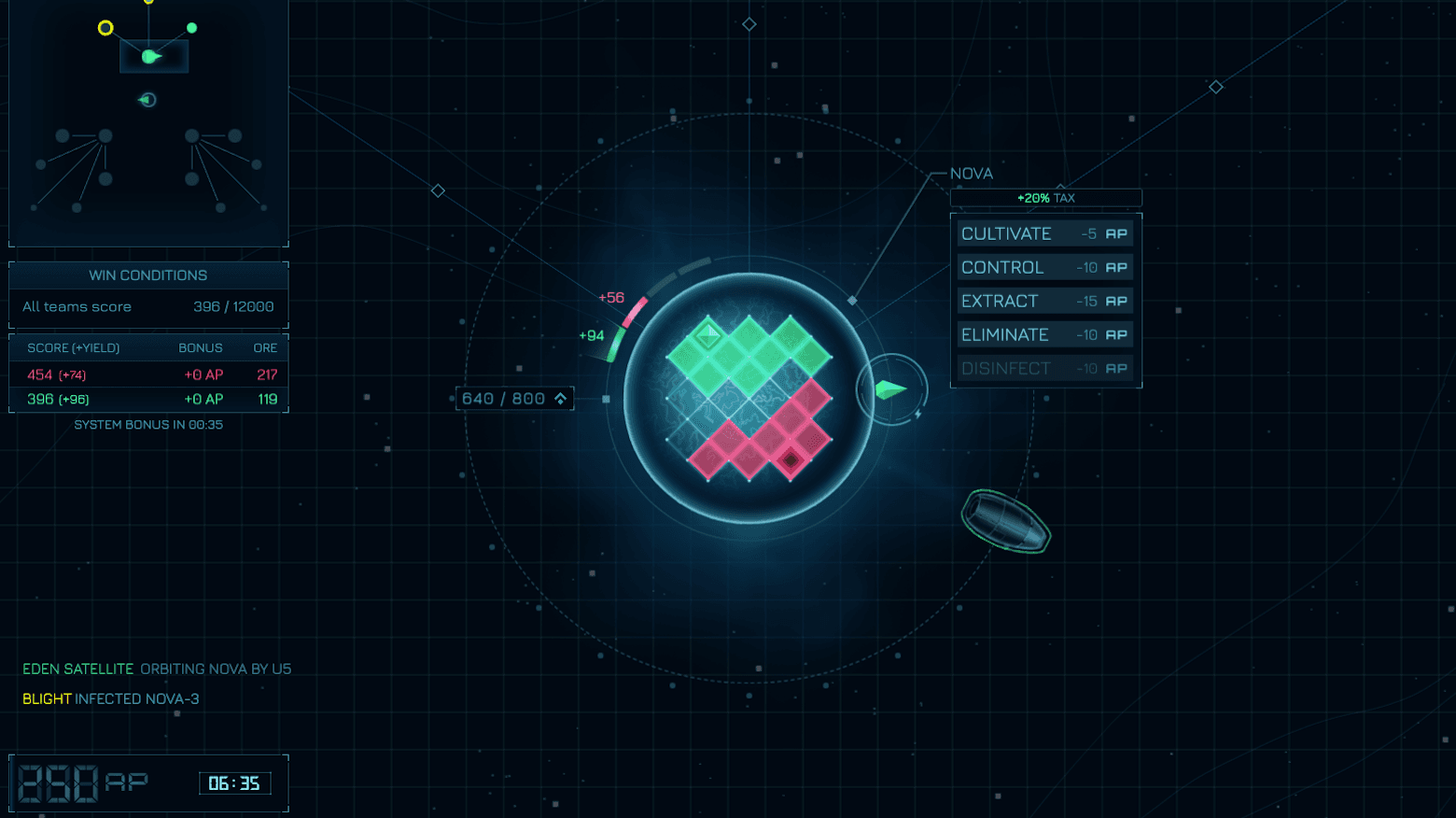
Proxima is a territory control game where the team needs to defend its intergalactic colony and conquer new planets.
Core Systems
- Designed and implemented a flexible game mode architecture:
- Implemented collaborative mode.
- Created a composable objective system allowing designers to produce a variety of new experiences.
- Built team-based scoring and objective-specific progress tracking.
interface ObjectiveHandler { sort(state: GameState, objective: Objective, a: Team, b: Team): number; hasBeenCompletedByTeam(state: GameState, objective: Objective, team?: Team): boolean; hasBeenCompleted(state: GameState, objective: Objective): boolean; getProgress(state: GameState, objective: Objective, team?: Team): number; getLabel(state: GameState, objective: Objective, team?: Team): string; getProgressLabel(state: GameState, objective: Objective, team?: Team): string; } // Map definition file { // ... gamemode: { collaborative: true, objectives: [ { type: 'ALL_TEAMS_SCORE', amount: 21000 }, { type: 'YIELD', amount: 1300 }, ], }, // ... }
- Implemented a game events system.
- Implemented an analytics system with game state snapshots.
- Implemented a player context system to track relevant gameplay data with flags, e.g:
hasSteered
,hasLeftHomePlanet
.
Platform and Performance
- Fixed source map support for better observability on the client monitoring service (Sentry).
- Improved performance by tweaking the rendering library defaults and enabling sprite culling.
Gameplay
- Built a new PvE mechanic:
- Spread when all the planet plots are infected.
- Active and Dormant states. Dormant activates when a player lands on the planet.
- Disinfect action and special satellite to counter.
- Visual effects and animations.
- Implemented a new planet resource (Ore):
- Added new Planet Plot type (Mine)
- Added new player action (Build Mine)
- Fixed ghost input on window blur with the key pressed.
- Implemented new satellites and side effects.
- Made pings available globally in collaborative scenarios.
- Implemented a context-sensitive tutorial system for FTUE.
UI
- Refactored the planet actions panel to be data-driven. Easier to add new actions.
const actions: Action[] = [ { label: BUILD_EXTRACTOR_BTN, tooltip: BUILD_EXTRACTOR_BTN_TOOLTIP, cost: EXTRACTOR_AP_COST, onClick: (planet: Planet) => { send('build', { planet_id: planet.id, type: BuildType.Extractor }) }, condition: (planet) => planet.plots.some((p) => p.team == null && !p.disabled), }, // ... ];
- Implemented custom data visualization components featuring:
- Line chart.
- Pie chart.
- Radar chart.
- Automatic resizing.
- Custom styling options.
- Labels.
- Auto-scaling based on the data set.
- Implemented an intro tutorial sequence.
- Implemented the satellite market interface.
- Added minimap tooltips.
AI
- Prototyped AI-driven spaceships:
- Preliminary work:
- Decoupled players from ships.
- Moved the simulation of some subsystems to server-side.
- Steering behaviors.
- Naive spatial sensor for level layout.
- 2D ray cast for collision detection.
- Behavior Tree.
- Preliminary work: