Hyperball
2D
TypeScript
Node.js
HTML5
Canvas
Colyseus
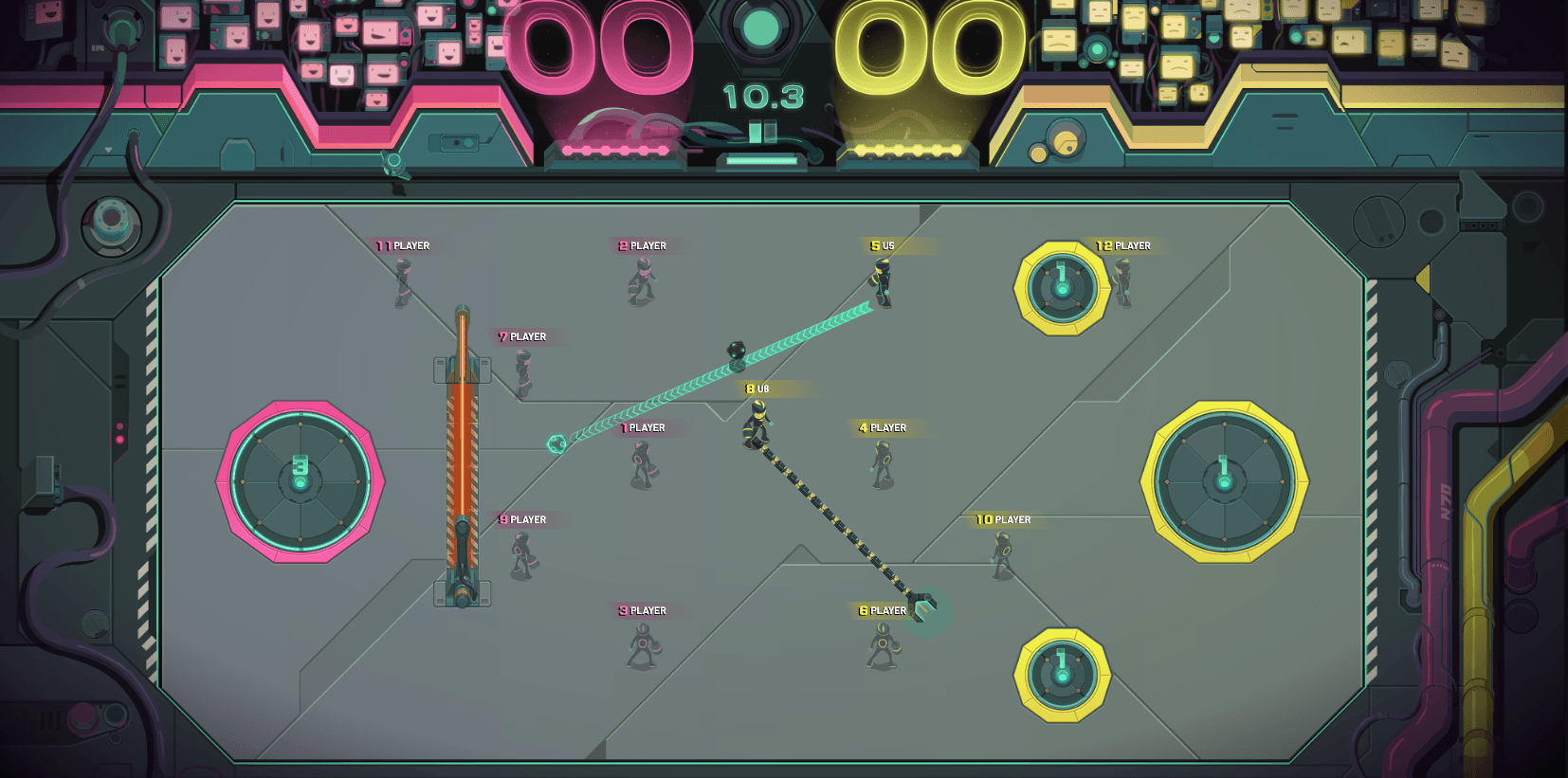
Hyperball is a turn-based, hyper-fast, futuristic sports simulation.
Technical Highlights
Core Systems
- Refactored the game initialization, adding explicit states.
- This fixed a critical network initialization issue. The game client attempted to connect to the server before loading all the assets. If the asset loading took too long, the server connection would be dropped due to heartbeat settings, leaving the client in a corrupted state.
enum InitializationState {
NONE,
LOADING_ASSETS,
CONNECT_TO_SERVER,
CONNECTING_TO_SERVER,
CONNECTED_TO_SERVER,
WAITING_FOR_MATCH_ACK,
RETRY,
}
- Refactored the core game loop, improving code readability and reliability.
- This fixed the "Halftime Bug," which existed longer than I was at the company. If your kids ever did Synthesis, they probably heard about this one.
- Exposed game and round length settings to designers.
- Tracked several new gameplay metrics.
- Implemented Canvas sprite tinting.
Platform
- Fixed source map support for better observability on the client monitoring service (Sentry).
- CI/CD tweaks, Dockerfile, and GitHub Actions.
- Strengthened platform integration by implementing missing event handlers: Name change, viewport size update, etc.
Gameplay
- Implemented the "Claw".
- A powerup that increases the range of players actions.
- Can be chained to create advanced strategies.
- Collaborated with designers to fine tune values for balancing.
- Integrated assets.
- Vector Math: atan2, normalizing, sum, subtraction, scaling, etc.
UI
- Implemented the loading screen.
- Implemented the "Claw" state UI.
Miscellaneous
- Implemented dynamic portal colors.
- As the levels' complexity increased, we needed more colors to indicate sibling portals.
- Adding more colors to the spritesheet was tedious and required unnecessary artist time.
- Used the newly implemented tinting system to assign portal colors dynamically.
const PORTAL_COLORS = [ '#c98b44', // ... ]; drawTintedSprite( // ... PORTAL_COLORS[portal.pairIndex % PORTAL_COLORS.length] // ... );